In the realm of the .NET ecosystem, background jobs are often an essential component of efficient and scalable applications. At the heart of this, the Hangfire library stands out with its combination of simplicity and power.
Understanding Hangfire's Core
Hangfire offers a multi-threaded, scalable solution to background processing. But its real value lies in its rich feature set:
- Persistent Job Storage: Jobs, even when not processed, are saved in a persistent store, ensuring no data loss.
- Real-Time Monitoring: Its web-based interface offers a real-time view of job states, allowing swift monitoring and management.
- Automatic Retries: Failures aren’t final. With Hangfire, jobs that fail can be automatically retried, ensuring that temporary glitches don’t disrupt the workflow.
Making Hangfire Work for You
Hangfire requires a bit of initial setup to integrate it into your .NET application properly, but once done, you get a robust background processing system. Let's break down the setup:
Configuring the Storage
Every job in Hangfire, whether scheduled, recurring, or executed, must be stored somewhere. Hangfire supports multiple storage options.
var connection = Configuration.GetConnectionString("HangfireConnection");
In the above line, we retrieve the connection string for our SQL Server database from the application's configuration.
Registering Hangfire Services
After setting up the connection, we must tell our application about Hangfire.
builder.Services
.AddHangfire(config => config.UseSqlServerStorage(connection));
Here, AddHangfire
registers the necessary services for Hangfire in our application's dependency injection container. The UseSqlServerStorage
method specifies that we are using SQL Server as our job storage.
Starting the Background Processing Server
We need to start Hangfire's background processing server to process the jobs. This component polls the job storage for pending jobs and executes them.
builder.Services.AddHangfireServer();
Note: You don't always need this in every application. For instance, you might have a separate service responsible for processing jobs and another just for enqueuing them. Only the processing service would require the AddHangfireServer()
call.
Accessing Hangfire's Dashboard
Hangfire's built-in web-based dashboard provides a real-time view of job states, histories, and more. Setup is as simple as including the following:
app.UseHangfireDashboard();
You can now access the Hangfire dashboard via the /hangfire
route in your application. It provides a comprehensive view of job activity and history, allowing easy monitoring and management. However, in a production environment, access to the dashboard should be restricted. Hangfire provides ways to set up authorization filters, ensuring only authorized personnel can access this insightful but sensitive information.
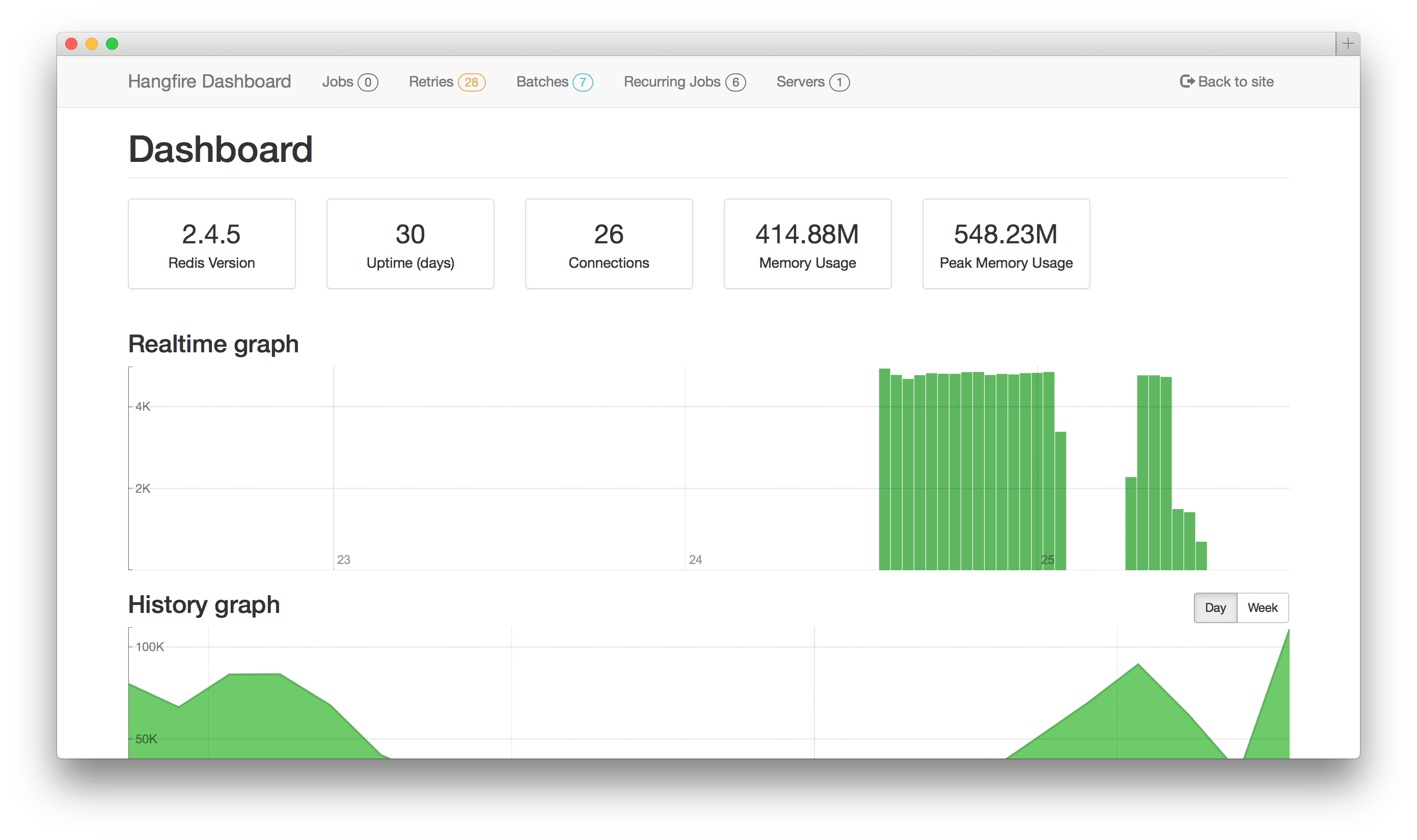
Leveraging Dependency Injection
One of Hangfire's strengths is its seamless integration with .NET Core's dependency injection system. This means your background jobs can easily use services registered in the DI container, such as database contexts, HTTP clients, etc. It streamlines the process of executing complex tasks that require various dependencies.
Storage Options
While we highlighted SQL Server as the job storage, Hangfire is versatile, supporting a range of storage mechanisms like Redis, MySQL, and more. The choice of storage can be tailored based on your application's demands and your operational environment.
Looking into Hangfire's Event Lifecycle
Hangfire has a rich event system, allowing developers to hook into various events, such as when jobs start, succeed, or fail. These can be invaluable for custom logging, notifications, or even triggering other business processes.
Scaling with Hangfire
As your application grows, Hangfire grows with it. Depending on the volume of background tasks, you can scale out Hangfire across multiple servers or increase the worker threads. This adaptability ensures that high loads are handled efficiently.
Utilizing the Extensions
The Hangfire ecosystem has a lot of extensions. Whether you're seeking additional dashboard views, specialized integrations, or unique job types, there's likely an extension out there to meet your needs.
Exception Handling and Troubleshooting
Beyond just automatic retries, Hangfire provides a detailed log of exceptions. This feature becomes valuable when troubleshooting failed jobs, giving developers a clear picture of what went wrong and where.
Using Hangfire Features
- Batch Operations: Handle bulk data operations in batches, optimizing performance.
var batchId = BatchJob.StartNew(job =>
{
for (var i = 0; i < 1000; i++)
{
job.Enqueue(() => Console.WriteLine($"Processing item {i}"));
}
});
- Scheduled Jobs: Plan ahead. Delay the execution or set up recurrent tasks with ease.
BackgroundJob.Schedule(
() => Console.WriteLine("Delayed job"),
TimeSpan.FromDays(1));
RecurringJob.AddOrUpdate(
() => Console.WriteLine("Recurring every day"),
Cron.Daily);
- Continuations: Chain tasks to ensure they run in the desired sequence.
var parentJobId = BackgroundJob.Enqueue(() => Console.WriteLine("First"));
BackgroundJob.ContinueJobWith(
parentJobId,
() => Console.WriteLine("Then this!"));
Concurrency Control and Job Execution in Hangfire
Beyond its facade, Hangfire uses a distributed locking mechanism to ensure that only one worker can process a job. Its intelligent scheduling system, combined with state-machine mechanics, guarantees the precision and reliability of background tasks. Managing concurrent job execution is a critical aspect of background task processing. Hangfire has mechanisms to help you, but understanding when and how to use them is important.
Built-in Concurrency Control
[DisableConcurrentExecution]: This attribute is a safeguard against the concurrent execution of the same job. Hangfire ensures a job isn't selected by multiple workers simultaneously. But for recurring jobs, there's no built-in prevention against the same job running in parallel if the previous instance hasn't finished. The attribute ensures that only one instance of a specific job type is in execution by leveraging a distributed lock. If the lock is not acquired, the job gets scheduled for a later retry.
Why Manual Locking?
Even with Hangfire's in-built mechanisms, there can be scenarios demanding a more granular control. For instance, recurring jobs with varied parameters are treated as distinct job types by Hangfire. So, if you have jobs targeting similar data but with different parameters, they might run concurrently. Implementing your own locking strategy, perhaps through a database lock or a distributed lock mechanism like Redis, can be invaluable here.
Recurring Job Gotchas
Recurring jobs are planned to run at fixed intervals. If an instance outlasts its scheduled interval, the following instance will still queue up. This can lead to concurrent runs of the same job. The [DisableConcurrentExecution]
attribute can aid in such situations, but for complex scenarios or jobs with varied parameters, a manual lock often becomes necessary.
In Conclusion
Hangfire is a great example of how elegance and functionality coexist in programming. When paired with the robustness of the .NET framework, it ensures your applications remain scalable, efficient, and agile.
Check out more details on the Hangfire web page.